Hi, I'm writing a plugin to dump out some additional stats for a load test (using OnLoadTestCompleted).
The plugin is working well but I would like to save the additonal data file to the same location as the websurge file.
Is there a way to access the session file path in a plugin?
stressTester and stressTester.options dont seem to expose this.
Thanks
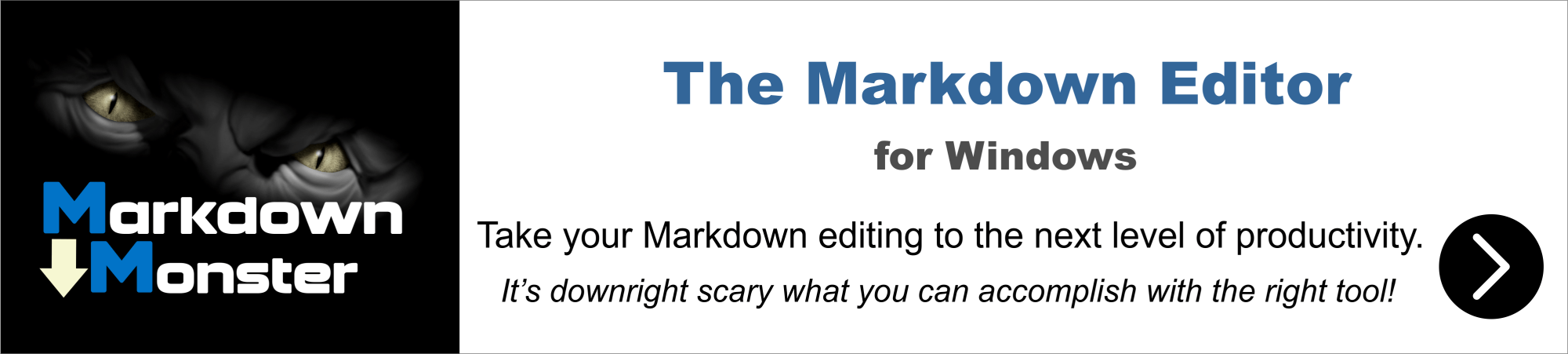
The StressTester is only a behavior object so it doesn't contain its own peristence information... that's stored in the UI model.
You can't get at that directly unfortunately because addins are tied to the core engine not the UI by default.
However, you can gain access to the UI and main AppModel by:
- Adding a reference to WebSurge.dll (the UI project)
- Using
AppModel.Current.FileName
You can capture the open file path here:
public override bool OnBeforeRequestSent(HttpRequestData httpRequest, StressTester stressTester)
{
httpRequest.AddHeader("rick-message",
"Hello World " + ShortDateString(DateTime.Now) );
var model = AppModel.Current;
var filename = model.FileName;
return true;
}
Note the filename could be empty if the Session has not been saved yet.
The AppModel also holds the open request list and also has a reference to the main Window - it's basically access to everything.
+++ Rick ---
Thanks Rick, thats done the trick!
For clarification:
By default a WebSurgeAddin doesn't include a reference to the WebSurge.dll
so that an addin can work in a non-UI environment. If running in the CLI for example, or if you hook the Core library into something else like a Web backend.
The filename probably should be stored somewhere in the addin but that would require some additional state information passed into the addin methods.
+++ Rick ---
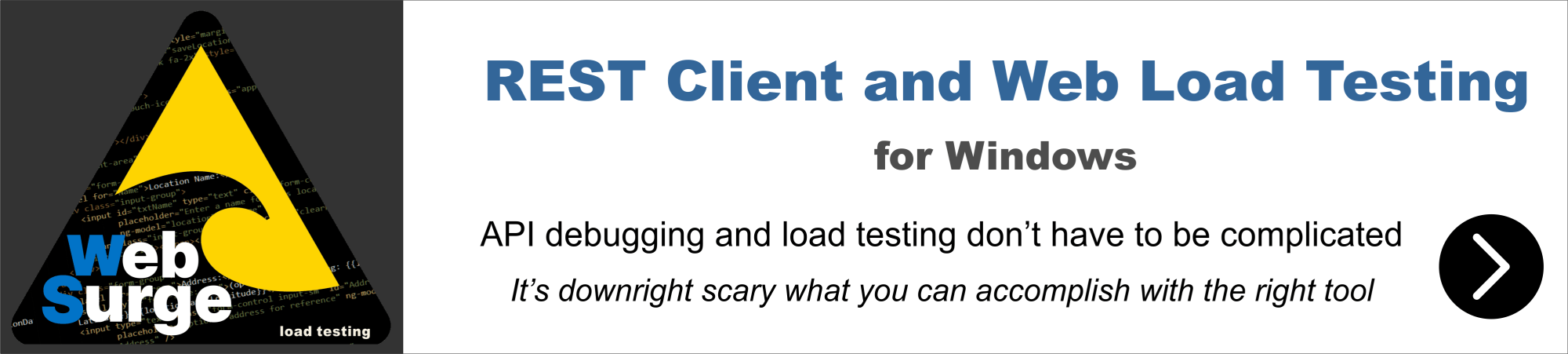
Thanks Rick, so does that mean this approach won't work for a test executed via the CLI?
No it won't work in the CLI because there's no UI project.
In the CLI you can use:
CommandLineOptions.SessionFile
Doing this so it works both in UI and CLI is tricky and would require Reflection with some error trapping.
Something like this:
public class SampleAddin : WebSurgeAddinBase
{
public string SessionFilename { get
{
string filename = null;
var model = ReflectionUtils.GetStaticProperty("WebSurge.AppModel", "Current");
if (model != null)
filename = ReflectionUtils.GetProperty(model, "FileName") as string;
if (string.IsNullOrEmpty(filename))
{
filename = ReflectionUtils.GetStaticProperty("WebSurge.Cli.CommandLineOptions", "SessionFile") as string;
}
return filename;
}
}
public override bool OnBeforeRequestSent(HttpRequestData httpRequest, StressTester stressTester)
{
httpRequest.AddHeader("rick-message",
"Hello World " + ShortDateString(DateTime.Now) );
var filename = SessionFilename;
return true;
}
}
+++ Rick ---
Hi Rick, stupid question, do I need to do something to enable addins for the CLI?
The addin using reflection is working fine in the UI but the addin doesnt seem to load/fire in the CLI.
Even if I hard code the path of my output file the CLI doesnt appear to recognize the addin.
Not that I know of - it should be working with the CLI just the same as in UI. Addins load during startup as part of the wsApp
initialization.
It's possible my syntax is wrong for the CLI filename but you can put in a Console.Write()
on load to see if that's firing.
I'll check tomorrow. I'm in the middle of doing a buch of updates in WS anyway, but haven't run the CLI in quite some time...
+++ Rick ---