FoxPro Programming
ChatGpt and VFP
There has been a lot of media about ChatGPT capabilities and several articles on its ability to program in any language. I did a quick search of this forum and found a request for a password checker. Here is what I asked of ChatGPT and the results.
*Write a visual foxpro function that will check passwords for strength. Return a numeric rating. *
FUNCTION CheckPasswordStrength(cPassword as String) as Integer
LOCAL nScore as Integer
nScore = 0
LOCAL nUpperCount as Integer
LOCAL nLowerCount as Integer
LOCAL nDigitCount as Integer
LOCAL nSpecialCount as Integer
LOCAL cChar as String
LOCAL nLength as Integer
LOCAL cLastChar as String
LOCAL lConsec as Logical
LOCAL lRepeat as Logical
LOCAL cAlphas as String
LOCAL cNumerics as String
LOCAL cSpecials as String
cAlphas = "abcdefghijklmnopqrstuvwxyz"
cNumerics = "01234567890"
cSpecials = "!@#$%^&*?_-"
nLength = LEN(cPassword)
* Check length
IF nLength > 7
nScore = nScore + 1
ENDIF
* Check for uppercase letter
nUpperCount = 0
FOR i = 1 TO nLength
cChar = SUBSTR(cPassword, i, 1)
IF ASC(cChar) >= 65 AND ASC(cChar) <= 90
nUpperCount = nUpperCount + 1
ENDIF
ENDFOR
IF nUpperCount > 0
nScore = nScore + 1
ENDIF
* Check for lowercase letter
nLowerCount = 0
FOR i = 1 TO nLength
cChar = SUBSTR(cPassword, i, 1)
IF ASC(cChar) >= 97 AND ASC(cChar) <= 122
nLowerCount = nLowerCount + 1
ENDIF
ENDFOR
IF nLowerCount > 0
nScore = nScore + 1
ENDIF
* Check for digits
nDigitCount = 0
FOR i = 1 TO nLength
cChar = SUBSTR(cPassword, i, 1)
IF ASC(cChar) >= 48 AND ASC(cChar) <= 57
nDigitCount = nDigitCount + 1
ENDIF
ENDFOR
IF nDigitCount > 0
nScore = nScore + 1
ENDIF
* Check for special characters
nSpecialCount = 0
FOR i = 1 TO nLength
cChar = SUBSTR(cPassword, i, 1)
IF !INLIST(cChar, cAlphas+cNumerics)
nSpecialCount = nSpecialCount + 1
ENDIF
ENDFOR
IF nSpecialCount > 0
nScore = nScore + 1
ENDIF
* Check for consecutive characters
cLastChar = ""
lConsec = .F.
FOR i = 1 TO nLength
cChar = SUBSTR(cPassword, i, 1)
IF cChar = cLastChar
lConsec = .T.
EXIT
ENDIF
cLastChar = cChar
ENDFOR
IF !lConsec
nScore = nScore + 1
ENDIF
* Check for repeating characters
lRepeat = .F.
FOR i = 1 TO nLength
cChar = SUBSTR(cPassword, i, 1)
IF OCCURS(cChar, cPassword) > 1
lRepeat = .T.
EXIT
ENDIF
ENDFOR
IF !lRepeat
nScore = nScore + 1
ENDIF
* Return the password strength rating
return nScore
ENDFUNC
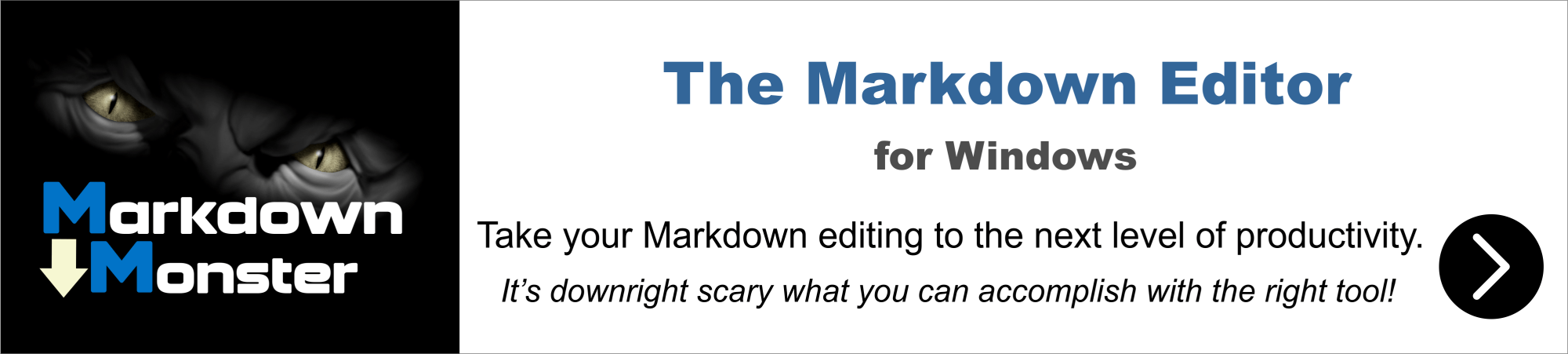
So - does that code actually work correctly? 😄
I played around with ChatGPT for a few things a while back and while I marvelled at the code it generated, it usually didn't get the job done. Unless you know what the code is supposed to do exactly and you can verify, you can't trust that code to be correct.
For me, the big plus is it can help with:
- Ideas of things to try
- A starting point for code
Just don't trust ChatGPT to get it right.
+++ Rick ---