Hello Rick and friends,
I need a password strength checker in Fox, would you think if I can use wwRegEx class?
And if yes, could you please let me know if there's an online example on how to use it?
Thank you in advance,
Kathy
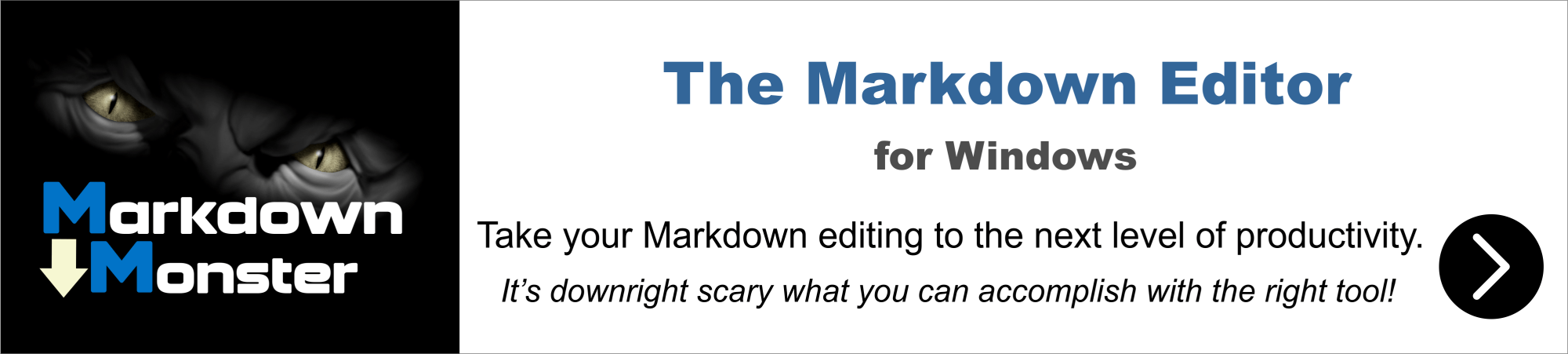
I would just manually write code for that.
Check for:
- Length (whatever you choose - I'd say 10 char minimum)
- Upper and Lower
- Number
- One Special Character
Whatever you do let people clearly know what's expected of your passwords otherwise it's a pain in the ass to guess...
+++ Rick ---
Thanks a lot Rick.
And if I want to use something like the following code in C# (found online) that gives scores to show the level of the strength, would you please advise on how to get the returned value by the function?
public enum PasswordScore
{
Blank = 0,
VeryWeak = 1,
Weak = 2,
Medium = 3,
Strong = 4,
VeryStrong = 5
}
public PasswordScore CheckStrength(string password)
{
int score = 0;
if (password.Length < 1)
return PasswordScore.Blank;
if (password.Length < 4)
return PasswordScore.VeryWeak;
if (password.Length >= 8)
score++;
if (password.Length >= 12)
score++;
if (Regex.Match(password, @"/\d+/", RegexOptions.ECMAScript).Success)
score++;
if (Regex.Match(password, @"/[a-z]/", RegexOptions.ECMAScript).Success &&
Regex.Match(password, @"/[A-Z]/", RegexOptions.ECMAScript).Success)
score++;
if (Regex.Match(password, @"/.[!,@,#,$,%,^,&,*,?,_,~,-,£,(,)]/", RegexOptions.ECMAScript).Success)
score++;
return (PasswordScore)score;
}
I'm not sure why I cannot see the function by:
loFox = loBridge.createinstance(...)
? loFox. && loFox doesn't show funciton CheckStrength!
And I'm not sure if/how I should use GetEnumValue, etc. for this case:
loBridge.GetEnumValue(...)
I would appreciate your advice on this.
Thanks,
Kathy
Is this your code (ie. you're compiling it)?
If so - change the signature to public static int
to remove the enum from the equation - you can cast the result to return (int) score
.
loBridge = GetwwDotnetBridge()
lnScore = loBridge.InvokeStaticMethod("YourNamespace.PasswordCheckerClass",CheckPassword,"seekrit#2")
public static int CheckPassword(string password)
{
var score = PasswordScore.Blank;
...
return (int) score;
}
+++ Rick ---
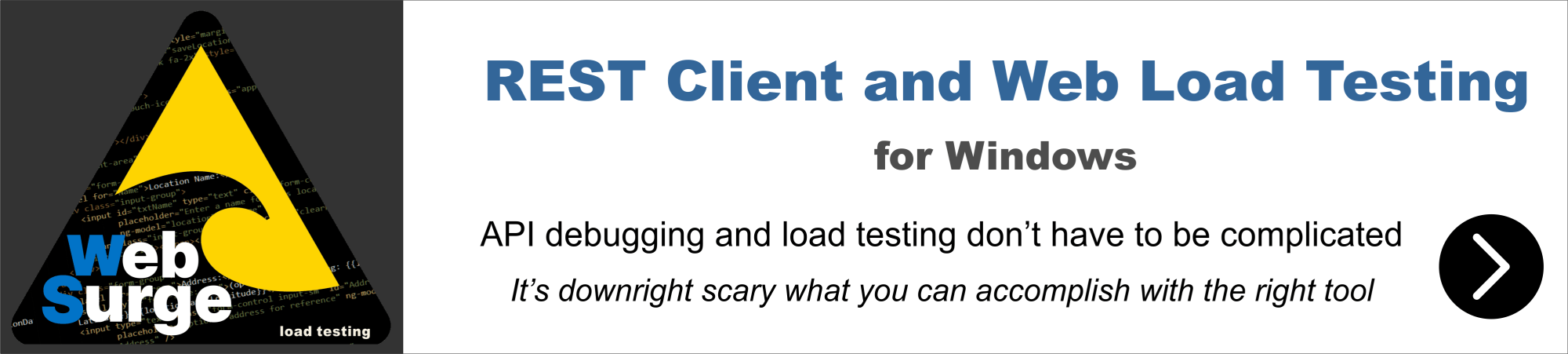