Hello
I have built a Web API endpoints using ASP.Net 5 to allow access to some COM object. I created a service that is responsible of creating new instance of the COM object and registered this service as Singleton. In my API controller I injected the service and from there I got access to COM object. The COM object has one object that has nested children objects of the same containing class type, as follows:
class Item
{
string id {get; set;}
string name {get; set;}
List<Item> Children {get; set;}
}
So I wrote an async recursive function to retrieve this object with all its nested children. I defined the function as follows:
[NonAction]
public async Task<Item> GetItem(string id){
var item = ComObj.getItem(id);
Item itm = new Item();
itm.Name = item.Name;
if (item.children.count > 0)
{
For(i=0;i<item.children.count;i++)
{
itm.Children.Add(await GetItem(item.children[i].Id));
}
}
return itm;
}
Now my problem is the performance is very bad, and it takes 4x the time of the same implementation if I run it from WinForm. So what is the best practice that can accelerate the performance?
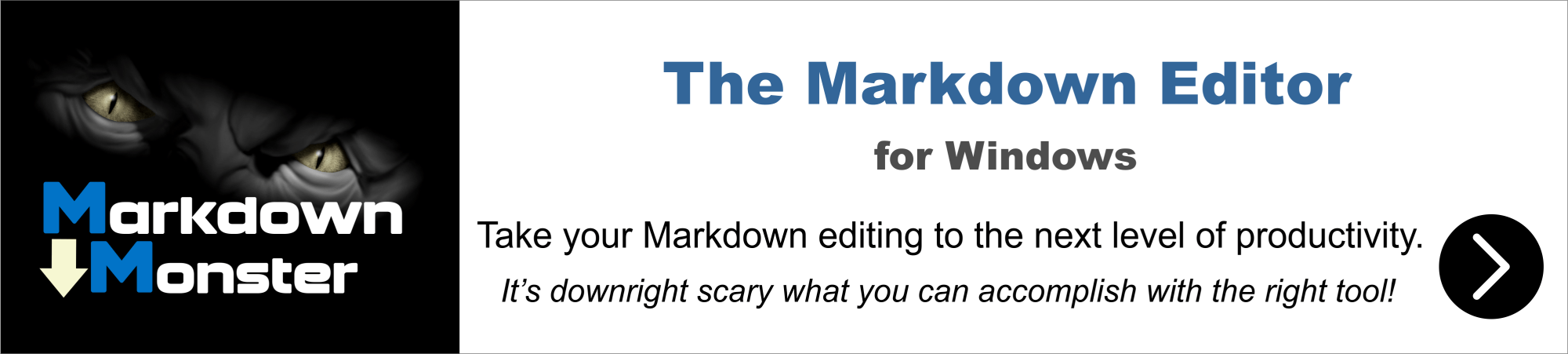
If you're doing STA COM interop in an ASP.NET Core application and you're not building a custom pool manager, it's not safe to use COM objects in this manner as .NET Core apps are purely MTA threaded. Running raw STA components in this way is not reliable - they will crash and corrupt when multiple instances are running simultanouesly.
The only way that you can make this work is through COM+ Component Services, or by building a custom thread manager for your objects and always delegating operations to the thread pool. COM+ will have significant overhead. A thread pool is a custom affair and won't let you call objects directly so it requires a lot of retooling, but it can provide good performance. That's what I use in Web Connection, but it's a fixed interface that always calls the same COM endpoints and handles the actual request processing inside of the executing component.
Unless you have a real good reason to use .NET Core I would suggest you don't use .NET Core for COM Interop, although you'd have the same problem if you use ASP.NET MVC (4 or prior) although there are workarounds for classic MVC. All these solutions don't support STA component access natively. The only Web technology that's STA friendly out of the box is WebForms or (with a little extra work) classic HTTP Handlers. Since you're running on Windows and with IIS there's little reason to use .NET Core unless there's a very specific reason to do so.
Or (if you're talking about FoxPro) you can use a tool like Web Connection which can work directly with .NET Core using the aforementioned thread pool approach where you preload instances and pass requests off to the pooled instances.
+++ Rick ---